Welcome, Guest |
You have to register before you can post on our site.
|
Forum Statistics |
» Members: 4,582
» Latest member: Lindabax
» Forum threads: 7,456
» Forum posts: 40,742
Full Statistics
|
|
|
Cannot Install BIMP to GIMP 3 |
Posted by: msindc - 6 hours ago - Forum: Extending the GIMP
- Replies (1)
|
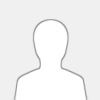 |
Folks:
When I try to add BIMP to GIMP, I get an error message that says:
"The installer cannot find GIMP installation by itself. Please click OK and manually select teh folder where GIMP is installed."
OK, fine. So I navigate to "C:\Program Files\Gimp 3" and select OK, but that triggers another error message:
"Error: Invalid GIMP plugins folder C:\Program Files\GIMP 3\lib\gimp\2.0\plug-ins."
The problem seems to be that BIMP is looking for C:\...gimp\2.0... but in my computer the folder is ...\3.0.
Is there any solution or workaround for this?
M.
|
|
|
Bug: gimp-drawable-get-pixel fails despite channels visible in UI |
Posted by: AgHornet - 8 hours ago - Forum: Scripting questions
- Replies (1)
|
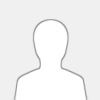 |
Anyone have any ideas?
Also, how is it possible that an RBG png image when opened in the GUI and image properties view, says it has 0 channels, but you can Go to Windows > Dockable Dialogs > Channels and select each of the 3 channels, np??
-----
**Bug Report Summary: gimp-drawable-get-pixel Returns UNEXPECTED_TYPE for Pixel Data Despite Channels Being Visible in UI**
**GIMP Version:** 2.10.38
**Operating System:** windows11
-----
**Description of Issue:**
When attempting to retrieve pixel data using the gimp-drawable-get-pixel PDB function within a Script-Fu plugin, the second return value (expected to be the GIMP_PDB_INT8ARRAY containing pixel bytes) is consistently an UNEXPECTED_TYPE from Script-Fu's perspective. This occurs even when the image clearly displays color channels in GIMP's UI.
**Detailed Observations:**
1. **Contradictory Channel Information:**
* Image > Image Properties for the affected image reports "Number of channels: 0".
* However, Windows > Dockable Dialogs > Channels *visibly shows* and allows interaction with R, G, and B channels (for an RGB image), and selecting/deselecting these channels correctly alters the image's display. This confirms that the channel data *does* exist internally and is usable by GIMP's UI.
2. **gimp-drawable-get-pixel Behavior:**
* The function call itself does not error out. It returns a Scheme PAIR (a two-element list) as expected.
* The first element of this pair (expected num-channels) appears to be accessible.
* The second element of this pair (expected pixel data, an INT8ARRAY or list of bytes) is the problem. Script-Fu's (cadr pixel-result) evaluates to a value that is not a list (empty or non-empty), boolean, number, string, or symbol. Our enhanced debugging in Script-Fu reports its type as UNEXPECTED_TYPE.
**Impact:**
This issue prevents Script-Fu plugins from reliably reading pixel data from images via gimp-drawable-get-pixel, effectively breaking any functionality that relies on pixel-level inspection (e.g., custom filters, image analysis, scene detection from masks).
**Steps to Reproduce:**
1. Open an RGB PNG image (e.g., scene_mask.png with visible white/black areas) in GIMP.
2. Verify the contradictory channel information:
* Go to Image > Image Properties and confirm "Number of channels: 0".
size in pixels: 7013 x 4062 pixels
Color space: RGB color: GIMP built-in sRGB
Precision: 8-bit gamma integer
File Type: PNG image
Number of pixels: 34798506
Number of layers: 1
Number of channels: 0
Number of Paths: 0
* Go to Windows > Dockable Dialogs > Channels and confirm that R, G, and B channels are visible and functional (e.g., toggling their visibility changes the image).
3. Load the Script-Fu plugin (with the relevant scan function snippet) into GIMP.
4. Run the Script-Fu plugin.
5. Observe the GIMP console or debug messages for output similar to:
script-fu.exe-Warning: Debug: Raw pixel-result at (X,Y): PAIR (Unknown Type)
script-fu.exe-Warning: Warning: pixel-bytes is not a valid list for pixel data at (X,Y). Raw value type: UNEXPECTED_TYPE Value: Cannot display raw value
**Expected Behavior:**
gimp-drawable-get-pixel should return a valid Scheme list of integer bytes (e.g., (255 255 255) for a white RGB pixel) as its second return value when invoked on a drawable from an image with visible and functional channels.
**Troubleshooting Performed (No Effect):**
* Attempted Image > Mode > Grayscale then Image > Mode > RGB.
* Attempted Image > Flatten Image and Image > Merge Visible Layers....
* Exported the image as a new PNG and then re-opened it in GIMP.
**Relevant Script-Fu Snippet (from scan function):**
scheme
(define (scan x y)
(let ((pixel-result (gimp-drawable-get-pixel mask-drawable x y)))
(gimp-message (string-append "Debug: Raw pixel-result at (" (number->string x) "," (number->string y) "): "
(if (pair? pixel-result) "PAIR" "NON-PAIR")
(if (and (not (pair? pixel-result)) (boolean? pixel-result)) (if pixel-result " (Value: TRUE)" " (Value: FALSE)")
(if (and (not (pair? pixel-result)) (number? pixel-result)) (string-append " (Value: " (number->string pixel-result) ")")
" (Unknown Type)"))))
(if (pair? pixel-result)
(let* (
(num-channels (car pixel-result))
(pixel-bytes (cadr pixel-result))
)
(if (and (pair? pixel-bytes) (not (null? pixel-bytes)))
(begin ; Process the pixel if valid
; ... (pixel processing logic) ...
)
(gimp-message (string-append "Warning: pixel-bytes is not a valid list for pixel data at (" (number->string x) "," (number->string y) "). Raw value type: "
(cond
((pair? pixel-bytes)
(if (null? pixel-bytes) "EMPTY_LIST ()" "NON_EMPTY_LIST"))
((boolean? pixel-bytes)
(if pixel-bytes "BOOLEAN_TRUE (#t)" "BOOLEAN_FALSE (#f)"))
((number? pixel-bytes) "NUMBER")
((symbol? pixel-bytes) "SYMBOL")
((string? pixel-bytes) "STRING")
(else "UNEXPECTED_TYPE"))
" Value: "
(cond
((boolean? pixel-bytes) (if pixel-bytes "#t" "#f"))
((number? pixel-bytes) (number->string pixel-bytes))
((pair? pixel-bytes) (if (null? pixel-bytes) "()" (string-append "(" (number->string (car pixel-bytes)) "...")) )
(else "Cannot display raw value")))))
)
(gimp-message (string-append "Error: gimp-drawable-get-pixel returned an unexpected value (not a pair) for pixel-result at (" (number->string x) "," (number->string y) "). Raw value: "
(if (boolean? pixel-result) (if pixel-result "TRUE" "FALSE") "Unknown type"))))
)
)
-----
|
|
|
Gimp 3.0.4 - Windows 10 - Always crash/terminates on loading image files |
Posted by: Grobe - 10 hours ago - Forum: Gimp 2.99 & Gimp 3.0
- Replies (1)
|
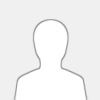 |
Hi.
Issue
Gimp initially seems to load normally (no files opened).
However, the following actions cause Gimp to crash and terminate without any error dialog box other than the Windows generic that just say the program has crashed and will now close.
- Drag an image file from windows explorer into Gimp canvas.
- Invoke the File --> Open dialog box, select an image file to open.
Information about Gimp installation
I don't if spending resources on a "dying OS" is worth the time. But here it goes anyway:
On my workplace, I use a W10 workstation. I have Gimp 2.10.38 and this version have no issues that I'd noticed.
Today I installed Gimp 3.0.4. The only specifics in installation is that
- Installation method: only for me
- Installation path: changed "GIMP 3" to "GIMP3"
- Languages: Only Norwegian (bokmål) and Canadian English.
OS information (Norwegian language) - manually filtering out sensitive info
Versjon Windows 10 Enterprise
Versjon 22H2
Installert den ...filter...
Operativsystembygg 19045.6036
Opplevelse Windows Feature Experience Pack 1000.19062.1000.0
Enhetsnavn ...filter...
Fullt enhetsnavn ...filter...
Prosessor Intel® Core i7-6700 CPU @ 3.40GHz 3.41 GHz
Installert RAM 8,00 GB (7,88 GB brukbar)
Enhets-ID ...filter...
Produkt-ID ...filter...
Systemtype 64-biters operativsystem, x64-basert prosessor
Penn og berøring Ingen penne- og berøringsinndata er tilgjengelige for denne skjermen
And then
Is this a known bug to Gimp 3.0.4? Link to bug report?
If no - should I post a bug repport for this.
And are there any log files, or way to open Gimp in such a way that errors get logged and that I can help the team ?
|
|
|
GIMP 3.04 opens with window extending below the taskbar on Windows 11 |
Posted by: SteveH - 07-01-2025, 11:47 PM - Forum: Windows
- Replies (3)
|
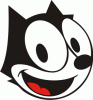 |
After installing GIMP 3.04 on Windows 11 Home 24H2 26100.4351, GIMP opens with the GIMP window extending below the taskbar and/or extending above the top of the screen. Also, when using View > Zoom > Fit Image in Window, the GIMP window changes to extending below the taskbar. Apparently there is a bug in Edit > Preferences > Interface > Windows Management > Save windows position on exit.
This is on 2 computers. Others have reported the bug: https://gitlab.gnome.org/GNOME/gimp/-/issues/14325
My solution with 2.10.38 already installed
- Backup my toolrc so I don't have to create it again.
- Delete the C:\Users\User_Name\AppData\Roaming\GIMP\3.0 folder.
- Open GIMP and have it create a new GIMP 3.0 folder structure and then close GIMP. It appears to copy some preferences and settings from 2.10.38 but not all.
- Create a default_rc folder and copy all rc files into it just to be safe.
- Copy my toolrc back into C:\Users\User_Name\AppData\Roaming\GIMP\3.0.
- Open GIMP and if it opens with the correct window size then:
- Fix the docked tab options, default grid spacing, default export file type and other preferences that did not copy over from 2.10.38.
- Adjust the canvas window size to fit a landscape oriented photo and adjust the docked window sizes.
- Edit > Preferences > Interface > Windows Management > Save window positions on exit = uncheck, click on Save Window Positions Now.
- Close GIMP.
This appears to fix the problem as the next day, GIMP opened with everything positioned correctly on both computers. I hope that this helps.
|
|
|
Missing script-fu "refresh scripts" submenu |
Posted by: Frenchie - 07-01-2025, 07:20 PM - Forum: Gimp 2.99 & Gimp 3.0
- Replies (11)
|
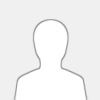 |
Hello,
Sorry for my bad English (translated by Google Translate),
I just downloaded version 3.0.4 of Gimp. Previously, I was using version 2.10.38.
I created several scripts that worked fine with version 2.10. Following the changes made in version 3.0, some functions have disappeared (for example, gimp-image-set-active-layer was replaced by gimp-image-set-selected-layers) or their parameters are different (for example, gimp-edit-copy has a new parameter "vector").
Updates are tedious (edit the script - test - edit again... until the script works correctly).
In version 2.10, it was possible to edit the script file (.scm) and have the update taken into account in Gimp via the "refresh scripts" submenu. However, this submenu is missing in version 3.0. You must therefore close Gimp and reopen it each time you make a change.
Do you know if there is a way to either make the "refresh scripts" submenu appear or to take script changes into account without closing Gimp?
Thank you in advance for your answers.
|
|
|
processes in Whitelist |
Posted by: Jean Dupont - 07-01-2025, 08:04 AM - Forum: OSX
- Replies (5)
|
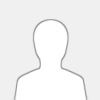 |
Hello,
I'm contacting you as part of the management of a macOS IT estate with an MDM (Mosyle), and I need to know which processes need to be on the application whitelist to be able to run GIMP and its features correctly?
Thank you very much
|
|
|
Can't see GIMP windows |
Posted by: Tankred - 06-30-2025, 12:12 AM - Forum: Gimp 2.99 & Gimp 3.0
- Replies (3)
|
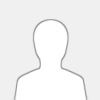 |
I have used GIMP for years. I upgraded to 3.0 and can't see any of the windows. I have it pinned to the taskbar ande tried to run it from there, nothing.
The services are running in Task Manager, both gdbus and GIMP. I deleted the sessionrc folder and restarted. I have reinstalled it many times. My graphics driver is up to date.
Here is what I have for my computer:
- Motherboard Name Asus ROG Maximus Z790 Dark Hero (1 PCI-E x4, 2 PCI-E x16, 5 M.2, 4 DDR5 DIMM, Audio, Video, 2.5GbE LAN, 2 Thunderbolt 4, WiFi)
- Video Adapter NVIDIA GeForce RTX 4080 SUPER (16376 MB)
- Operating System Microsoft Windows 11 Pro
and various normal things.
I am a total loss because I have never seen this and I am a computer technician/cybersecuritry analyst.
Please help as I have been thru almost every suggestion on Google!
Thanks
|
|
|
clone tool |
Posted by: honeybee2003 - 06-28-2025, 05:15 PM - Forum: General questions
- Replies (6)
|
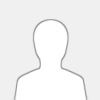 |
hi,
I click the pic to set up the area I want colour to clone from
I go over and start changeing the pic
the spot Im getting colour from keeps moving
Is there any way to make this fixed and get colour from one exact location ?
thanks for help or comments....
|
|
|
|