Welcome, Guest |
You have to register before you can post on our site.
|
Latest Threads |
edit_paste pastes at inco...
Forum: Scripting questions
Last Post: class43
8 hours ago
» Replies: 2
» Views: 116
|
CMYK color mode Gimp 2.10
Forum: Extending the GIMP
Last Post: rich2005
Yesterday, 12:13 PM
» Replies: 20
» Views: 82,185
|
Open, save buttons on bot...
Forum: Gimp 2.99 & Gimp 3.0
Last Post: GrumpyDeveloper
07-17-2025, 09:52 PM
» Replies: 8
» Views: 2,953
|
Clone size randomly chang...
Forum: General questions
Last Post: oldschool1@runbox.com
07-17-2025, 05:45 PM
» Replies: 2
» Views: 188
|
AI Gimp Plugins
Forum: Watercooler
Last Post: Zbyma72age
07-17-2025, 03:31 PM
» Replies: 20
» Views: 53,547
|
Gimp closes automatically
Forum: General questions
Last Post: sallyanne
07-17-2025, 05:50 AM
» Replies: 2
» Views: 217
|
AIGoR - Artificial Image ...
Forum: Other graphics software
Last Post: vitforlinux
07-16-2025, 11:10 AM
» Replies: 12
» Views: 3,839
|
Is This Possible ? Print ...
Forum: General questions
Last Post: sallyanne
07-16-2025, 07:47 AM
» Replies: 4
» Views: 208
|
Gimp Crash
Forum: Gimp 2.99 & Gimp 3.0
Last Post: rich2005
07-16-2025, 07:16 AM
» Replies: 1
» Views: 153
|
producing an image
Forum: Gallery
Last Post: MJ Barmish
07-15-2025, 06:37 PM
» Replies: 0
» Views: 114
|
|
|
I cannot find the "heal-selection-tool" |
Posted by: joppla - 10-16-2023, 08:25 PM - Forum: General questions
- Replies (2)
|
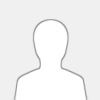 |
Hello,
I am not very familiair to GIMP, but I hope somebody can help me.
I use Ubuntu 20.04
I had GIMP 2.10.18
I installed the Resynthsizer plugin
I see now f.e. FX-foundry and Script-Fu
In the map with plugins I see the heal-selection-tool.py and other files from resynthesizer
But when I go to Filters>Enhance> I cannot find the heal-selection-tools that there should be.
That's why I updated Gimp from 2.10.18 to 2.10.34.
But still I cannot find the tool.
I is it replaced to another location? Or is there a setting that I missed anywhere?
Thanks for a reaction
|
|
|
angle of a path information ? |
Posted by: denzjos - 10-13-2023, 11:42 AM - Forum: General questions
- Replies (8)
|
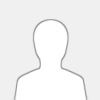 |
I've traced some straight paths on an existing map. Is there a plugin that shows the angle of each of the lines, referring to zero degrees or 90 degrees? The gimp plugin 'Path Details' only provides the path length and number of points.
|
|
|
Disabling "auto-select" |
Posted by: gt40mk2 - 10-11-2023, 08:51 PM - Forum: General questions
- Replies (4)
|
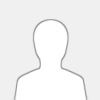 |
Hi - I'm a PS user, but can no longer justify the cost. I'm new to Gimp, just figuring out the basics.
Mac OS 10.13, Gimp 2.10.34 rev 3
How do I make it *not* auto-select (as it's called in PS)?
eg: I select a layer in the layer list. When I click on the screen to move it, it will move what is under the cursor on the screen, or the topmost layer above the selected layer on the screen.
I tried Prefs > Tool Options > Set layer or path as Active - both on and off - it doesn't make a difference.
Thanks!
|
|
|
32 bit images, Alpha channel, API Win32. |
Posted by: todmar - 10-10-2023, 01:30 AM - Forum: Windows
- Replies (3)
|
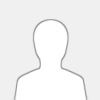 |
Exporting a .bmp file in 32 bit from Gimp: the image does not work on Windows. (LoadImage() call)
Load and save in other programs fixes the bug.
(such as Pixelformer)
The way GIMP does Color Alpha Transparent and Opaque is way cool.
|
|
|
Centering text |
Posted by: sl60 - 10-09-2023, 08:11 PM - Forum: General questions
- Replies (7)
|
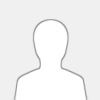 |
I'm having a problem centering text layers. I select the text>Alignment Tool>Center Icon--and nothing. Same with Justify. What am I doing wrong?
|
|
|
Can't get resynthesizer to work on Gimp 2.10.34 |
Posted by: giutor - 10-08-2023, 10:31 AM - Forum: Linux and other Unixen
- Replies (6)
|
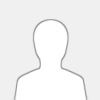 |
Hi,
I downloaded the plugin from the github repository but I get this ouput:
Code:
Parsing '/home/gt/.config/GIMP/2.10/pluginrc'
Querying plug-in: '/usr/lib/gimp/2.0/plug-ins/file-rawtherapee/file-rawtherapee'
Querying plug-in: '/usr/lib/gimp/2.0/plug-ins/file-darktable/file-darktable'
Querying plug-in: '/home/gt/.config/GIMP/2.10/plug-ins/plugin-uncrop.py'
File "/home/gt/.config/GIMP/2.10/plug-ins/plugin-uncrop.py", line 94
raise RuntimeError, "Failed duplicate image"
^
SyntaxError: invalid syntax
gimp: LibGimpBase-WARNING: gimp: gimp_wire_read(): error
Terminating plug-in: '/home/gt/.config/GIMP/2.10/plug-ins/plugin-uncrop.py'
Querying plug-in: '/home/gt/.config/GIMP/2.10/plug-ins/plugin-resynth-sharpen.py'
File "/home/gt/.config/GIMP/2.10/plug-ins/plugin-resynth-sharpen.py", line 53
raise RuntimeError, "Failed duplicate image"
^
SyntaxError: invalid syntax
gimp: LibGimpBase-WARNING: gimp: gimp_wire_read(): error
Terminating plug-in: '/home/gt/.config/GIMP/2.10/plug-ins/plugin-resynth-sharpen.py'
Querying plug-in: '/home/gt/.config/GIMP/2.10/plug-ins/plugin-resynth-fill-pattern.py'
Traceback (most recent call last):
File "/home/gt/.config/GIMP/2.10/plug-ins/plugin-resynth-fill-pattern.py", line 33, in <module>
from gimpfu import *
ModuleNotFoundError: No module named 'gimpfu'
gimp: LibGimpBase-WARNING: gimp: gimp_wire_read(): error
Terminating plug-in: '/home/gt/.config/GIMP/2.10/plug-ins/plugin-resynth-fill-pattern.py'
Querying plug-in: '/home/gt/.config/GIMP/2.10/plug-ins/plugin-resynth-enlarge.py'
File "/home/gt/.config/GIMP/2.10/plug-ins/plugin-resynth-enlarge.py", line 54
raise RuntimeError, "Failed duplicate image"
How do I get Resynthesizer to work? Thanks
|
|
|
|